While doing a JavaScript exploit I encountered that there isn’t a convenient function in JavaScript to set specific code to a specific offset in a given string.
For example:
Given the string “1234567890”
If you want to change the substring “567” to “756” for it to become “1234765890”
you would need to do something like this:
var string = “1234567890”;
var LeftHalf = String.substr(0 , 3); // leftHalf = “1234”
var RightHalf = String.substr(3); // RightHalf = “56789”
RightHalf = RightHalf.substr(3); // RightHalf = “89” (remove "567")
String = LeftHalf + “765” + RightHalf; // String = “1234765890”
Of course you could’ve just make a new string with the desired changes, but when you have a very long string and you’re making multiple modifications to different offsets of it, like you probably would making an exploit, it can become a tedious pain.
So to make this more consistent, time efficient, and less likely to make mistakes, I created a general function so you can modify your string with a single line.
function SetOffset(String, Offset, Injection)
{
var LeftHalf = String.substr(0 , Offset);
var RightHalf = String.substr(Offset);
RightHalf = RightHalf.substr(Injection.length);
var ModifiedString = LeftHalf + Injection + RightHalf;
return ModifiedString;
}
With this new function, the above piece of code would become:
var String = “1234567890”
String = SetOffset(String, 3, “765”);
This code works perfectly with the Metasploit tools “Pattern_Create.rb” and “Pattern_Offset.rb”. You just need to copy this function somewhere in the beginning of your code and you can call it as needed.
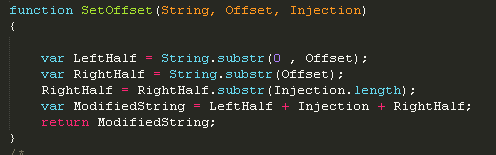